Build a Blog Application with Next.js 14: A Step-by-Step Tutorial
In this tutorial, we’ll build a fully functional blog application using Next.js 14, leveraging its newest features for dynamic routing, SEO, and performance optimization. This project is designed to help you learn practical Next.js 14 concepts step-by-step.
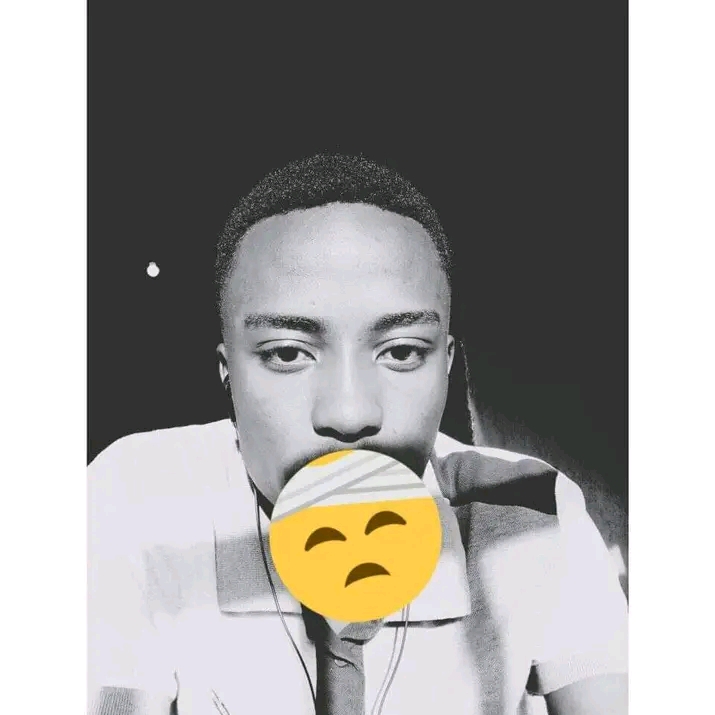
Peter Nzana
Lecture 3 min
Publié le 24 novembre 2024
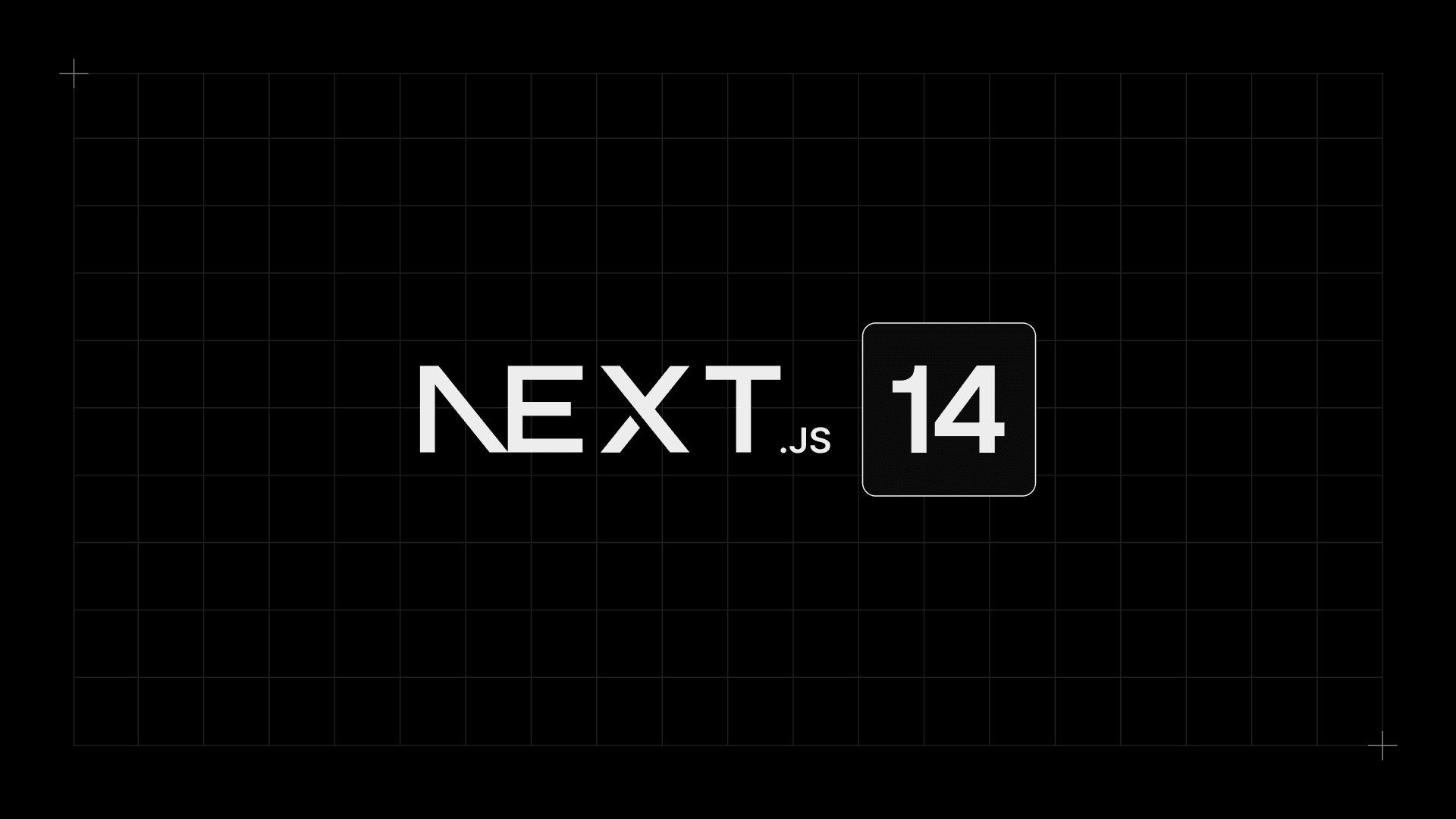
Table of Contents
Project Overview
In this project, we’ll build a dynamic blog application with the following features:
-
Dynamic routes for blog posts.
-
Real-time fetching of blog content from a mock API.
-
SEO-optimized pages using Next.js 14 metadata tools.
-
Responsive design with CSS modules.
By the end of this tutorial, you will have a deployable blog application.
Setting Up the Environment
-
Install Node.js Ensure you have Node.js version 18 or higher installed on your system.
-
Initialize the Project Run the following command to set up a new Next.js project:
npx create-next-app@latest blog-app
cd blog-app
- Start the Development Server Launch the server to view the app:
npm run dev
Open your browser and navigate to
http://localhost:3000.
Creating a Dynamic Blog App
- Setting Up the Blog Structure
Create a folder for the blog pages under the app directory:
mkdir -p app/blog/[slug]
- Dynamic Routing with App Router
Create a dynamic route for blog posts:
// app/blog/[slug]/page.js
import { use } from 'react';
async function fetchPost(slug) {
const res = await fetch(`https://jsonplaceholder.typicode.com/posts/${slug}`);
if (!res.ok) throw new Error('Post not found');
return res.json();
}
export default function BlogPost({ params }) {
const post = use(fetchPost(params.slug));
return (
<article>
<h1>{post.title}</h1>
<p>{post.body}</p>
</article>
);
}
Key Concepts:
Dynamic Routing: [slug] allows the app to handle different URLs dynamically.
Server-Side Rendering: use(fetchPost) fetches data on the server for better SEO.
- Fetching Blog Posts
To display a list of blog posts, create an index page:
// app/blog/page.js
import Link from 'next/link';
async function fetchPosts() {
const res = await fetch('https://jsonplaceholder.typicode.com/posts');
return res.json();
}
export default async function Blog() {
const posts = await fetchPosts();
return (
<section>
<h1>Blog Posts</h1>
<ul>
{posts.map((post) => (
<li key={post.id}>
<Link href={`/blog/${post.id}`}>{post.title}</Link>
</li>
))}
</ul>
</section>
);
}
- Styling the Application
Add styles using CSS Modules:
/* styles/blog.module.css */
.container {
font-family: Arial, sans-serif;
margin: 0 auto;
max-width: 800px;
padding: 1rem;
}
h1 {
color: #333;
}
Import the styles in your components:
import styles from './styles/blog.module.css';
export default function Blog() {
// Use `styles.container` for styling
}
Adding SEO Optimization
Use the Metadata API to add SEO tags to the blog:
export const metadata = {
title: 'Dynamic Blog App | Next.js 14',
description: 'A fully functional blog app built with Next.js 14.',
};
For individual blog posts, generate dynamic metadata:
export async function generateMetadata({ params }) {
const post = await fetchPost(params.slug);
return {
title: `${post.title} | Blog`,
description: post.body.substring(0, 150),
};
}
Deploying the Application
- Install Vercel CLI:
npm install -g vercel
- Deploy the App: Run the following command and follow the prompts:
vercel
- Live URL: Your app will be live at a unique URL provided by Vercel.
Resources
Last updated: 24 novembre 2024